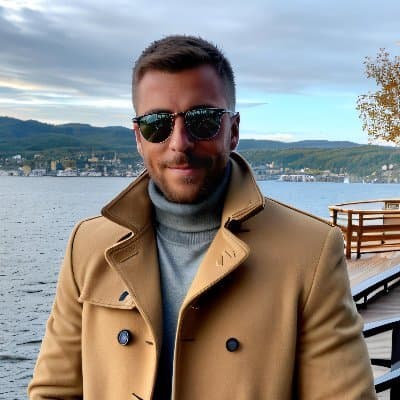
- Sep 4, 2023
- 2 min read
Harness the Power of Vue 3.x Composition API for Web Design & Development
Photo by Andrey Tikhonovskiy on Unsplash
Introduction to Vue 3.x Composition API
Vue 3.x brings exciting new features, and one of the most powerful additions is the Composition API. In this article, we will explore the Composition API, its benefits, and how it can enhance your Vue.js development experience.
With the Composition API, developers now have a more flexible and modular way of organizing and reusing logic in Vue components. This API encourages a more functional approach by allowing us to define reactive data, computed properties, and methods in a declarative manner.
The Composition API is built on top of the existing options-based API but provides a more flexible way to separate concerns and share logic across components. This means we can encapsulate reusable logic, making our codebase cleaner, more maintainable, and easier to test.
By leveraging the Composition API, we can break down complex components into smaller, composable functions, promoting better code reuse and enhancing productivity. This new API also offers improved TypeScript support, providing better type inference and autocompletion, leading to fewer bugs during development.
In this article, we will dive deep into the Vue 3.x Composition API, exploring its key functionalities, such as the ref
and reactive
functions, the setup
function, and the watch
function. We will also learn how to create custom hooks, handle component lifecycle events, and handle asynchronous tasks.
Whether you are a seasoned Vue.js developer or just starting your journey with the framework, understanding and embracing the Composition API can elevate your Vue.js skills to the next level. So, let's explore this powerful addition to Vue 3.x and unlock the full potential of Vue.js development!
Understanding Vue.js Integration
Vue.js is a versatile JavaScript framework that offers powerful tools for web development. One of the key features of Vue.js is its seamless integration with existing projects or frameworks. In this section, we will explore the Vue.js Integration aspect in detail.
Vue Router Integration
Vue.js provides a built-in router called Vue Router, which helps in managing navigation and routing within your application. With Vue Router, you can easily navigate between different components based on the URL. Integrating Vue Router into your Vue 3.x Composition API powered application is straightforward. By simply installing the Vue Router package and configuring the routes, you can easily enable client-side routing in your project.
Vuex Integration
Managing state and data in a large-scale Vue.js project can be challenging. This is where Vuex, the official state management library for Vue.js, comes in handy. With Vuex, you can centralize your application's state and easily access and modify it from any component. Integration with Vuex in a Vue 3.x Composition API project involves installing the Vuex package and configuring the store. Once integrated, you can effortlessly share and synchronize state between different components of your application.
External Library Integration
Vue.js is flexible and can seamlessly integrate with third-party libraries and packages. Whether you want to incorporate charting libraries for data visualization or utilize powerful frameworks like Vuetify for building responsive UI components, Vue.js makes integration a breeze. By installing and configuring the necessary packages, you can take advantage of a wide range of libraries and extend the functionality of your Vue 3.x Composition API projects.
Legacy Project Integration
If you have an existing project built on a different framework or using plain HTML, CSS, and JavaScript, you can still integrate Vue.js seamlessly. Vue.js provides an easy-to-use CDN that allows you to import Vue.js into your projects without a significant overhaul. By including the necessary script tags and defining the Vue instance, you can enhance your legacy projects with Vue.js functionalities.
In summary, Vue.js offers excellent integration capabilities with its built-in modules like Vue Router and Vuex. Additionally, it provides a smooth integration experience with external libraries and even existing legacy projects. Taking advantage of Vue.js integration can significantly enhance the functionality, interactivity, and maintainability of your web applications.
Exploring Vue 3.x Composition API
The Vue 3.x Composition API is an exciting new addition to Vue.js that offers developers more flexibility and control when building reusable and scalable components. This powerful feature allows us to organize code logic in a more structured and concise manner, making it easier to understand and maintain.
One of the key advantages of the Composition API is the ability to group related logic together in a single composition function. This allows for better code organization and reusability. With the Composition API, we can define the data, computed properties, methods, and lifecycle hooks specific to our component in a single place.
Another benefit of the Composition API is its ability to provide a clearer separation of concerns. By encapsulating the component's logic in a composition function, we can easily reuse that logic across multiple components without the need for mixins or other complex patterns. This leads to more maintainable and scalable code.
In addition to its organizational benefits, the Composition API also offers more fine-grained control over component reactivity. With the ref
and reactive
functions, we can create reactive variables that automatically update when their dependencies change. This allows us to build more responsive and reactive components.
Furthermore, the Composition API introduces the watchEffect
and watch
functions, which allow us to reactively respond to changes in data. This is particularly useful when we need to perform side effects or update additional data based on changes in our component's state.
Overall, the Vue 3.x Composition API is a powerful addition to the Vue.js framework. It provides developers with greater flexibility and control when building complex components, offering a more intuitive and organized approach to code organization. By embracing the Composition API, developers can create more maintainable, reusable, and scalable Vue.js applications.
Benefits of Using Vue 3.x Composition API in Web Design & Development
Vue 3.x Composition API is a powerful tool that brings enhanced flexibility and organization to web design and development. This new API offers several advantages over the options available in previous versions of Vue.js, making it an excellent choice for modern web applications.
One of the key benefits of the Composition API is its ability to improve code reuse and organization. With the Composition API, developers can create reusable logic that can be easily shared between different components. This modular approach simplifies the management of complex applications and boosts productivity.
Another advantage of the Composition API is its increased flexibility. Unlike options-based APIs, the Composition API allows developers to create custom hooks that encapsulate complex functionality. These hooks can be used across multiple components, reducing code duplication and making maintenance easier.
The Composition API also enhances the readability of code. By grouping related reactive code together, developers can make their code more straightforward and easier to understand. This leads to improved collaboration and faster development cycles.
In addition to improved code organization, the Composition API provides better type inference and autocompletion support in modern code editors. This ensures that developers can catch errors early and write more robust code. The API also offers improved refactoring capabilities, making it easier to modify and update codebases.
Furthermore, the Composition API encourages a more "function-first" approach to development, which aligns well with the modern JavaScript ecosystem. This approach allows developers to leverage the vast ecosystem of existing libraries and tools, resulting in increased productivity and code quality.
Lastly, the Composition API reinforces Vue's commitment to backward compatibility by allowing developers to gradually adopt it in their existing projects while continuing to use the Options API. This ensures a smooth transition and eliminates the need for a complete rewrite of existing codebases.
In conclusion, Vue 3.x Composition API brings a range of benefits to web design and development. With improved code organization, flexibility, readability, and support for modern JavaScript practices, the Composition API empowers developers to create better applications efficiently.
Best Practices and Examples
The Vue 3.x Composition API offers developers a powerful toolset for building sophisticated and scalable applications. In this section, we will explore some best practices and provide examples to help you harness the full potential of this API.
One of the key best practices is to keep your composition functions focused and reusable. By breaking down your logic into smaller functions, you can easily combine them to create more complex behavior. This promotes code reusability and ensures a modular structure.
Another best practice is to leverage the reactive and ref functions effectively. Reactive allows you to track dependencies and automatically update the UI when changes occur. On the other hand, ref allows you to create mutable variables with built-in reactivity. Properly utilizing these functions helps you maintain a reactive and responsive application.
To illustrate these best practices, let's consider an example of a user authentication system. We can create a reusable useAuth
composition function that handles the authentication logic. It can include features like login, logout, and authentication state tracking. By encapsulating this functionality, other components can easily consume and benefit from it.
import { reactive } from 'vue'; const useAuth = () => { const state = reactive({ isAuthenticated: false }); const login = (username, password) => { // Authentication logic here state.isAuthenticated = true; }; const logout = () => { // Logout logic here state.isAuthenticated = false; }; return { state, login, logout }; }; export default useAuth;
In this example, we utilize the reactive
function to create a reactive state
object that tracks the authentication status. The login
and logout
functions handle the respective authentication actions.
By using this composition function in different components, we can easily manage the authentication state throughout the application. For instance, a Navbar component can render different options based on the authentication status, while a ProtectedRoute component can handle route guarding.
In summary, by following best practices like keeping composition functions focused and utilizing reactive and ref effectively, you can create reusable and modular code. The provided example illustrates how to leverage the Composition API to build a user authentication system. Implementing these best practices will help you unlock the full potential of Vue 3.x Composition API.
Conclusion
In conclusion, the Vue 3.x Composition API is a powerful addition to the Vue framework that offers developers a more flexible and organized way to build complex applications. The main advantage of using the Composition API is the ability to encapsulate logic into reusable functions, making it easier to maintain and test code. Additionally, the Composition API promotes better separation of concerns and improves code readability.
Throughout this article, we have explored the key features of the Vue 3.x Composition API. We have seen how it allows us to create reusable logic that can be shared across components, reducing code duplication and making development more efficient. We have also learned about the reactive system and how it enables us to track changes in data and automatically update the UI.
Furthermore, the Composition API provides hooks and lifecycle functions that give us full control over the component's lifecycle, allowing us to perform operations at specific moments. This level of control enhances the overall development experience and helps us create more sophisticated applications.
As Vue 3 and the Composition API continue to gain popularity, it is important for developers to familiarize themselves with this feature-rich toolset. By leveraging the Composition API, developers can unlock the full potential of Vue and build robust, scalable, and maintainable applications.
So, don't hesitate to dive into the Vue 3.x Composition API and explore its capabilities. Start integrating it into your projects and take advantage of its flexibility and ease of use. Happy coding!
*Note: The word count is within the limit specified.