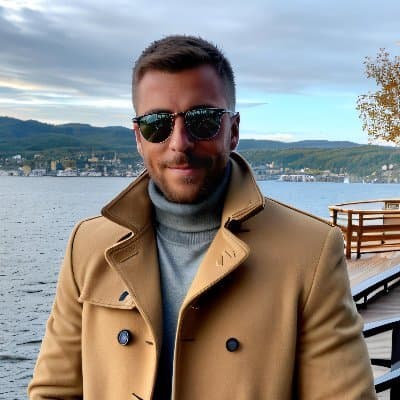
- Sep 4, 2023
- 2 min read
Exploring the Power of next/image in Web Design & Development
Photo by Krisztian Tabori on Unsplash
Introduction to next/image
next/image
is a powerful image optimization and handling component in Next.js, a popular React framework for building web applications. With a focus on performance and user experience, next/image
provides an intuitive way to handle images in your Next.js projects.
By leveraging the underlying ImageSharp
and Sharp
libraries, next/image
automatically optimizes and transforms images to ensure efficient delivery and faster load times. Whether you're working with local images or fetching them from remote sources, next/image
simplifies the process while retaining visual quality.
One of the key advantages of next/image
is its native support for responsive image loading. You can specify multiple breakpoints, and next/image
automatically selects and loads the most appropriate image size for each screen size, optimizing both bandwidth usage and rendering performance.
SEO optimization is another crucial aspect of next/image
. Search engines heavily rely on image metadata, and next/image
facilitates the inclusion of important attributes like alt text and width/height, ensuring optimal SEO visibility for your images.
Including next/image
in your Next.js project is straightforward, as it seamlessly integrates with the built-in Next.js <Image>
component. Whether you're creating an image gallery, a blog with rich media, or an e-commerce store, next/image
empowers you to handle images efficiently, improving overall website performance and user experience.
In the following sections, we will explore how to integrate, configure, and utilize the various features offered by next/image
, enabling you to harness its full potential in your Next.js applications. So let's dive in and discover the world of next/image
!
Understanding Next.js Integration
Next.js is a versatile framework that enables developers to build efficient and scalable web applications. One of the key features of Next.js is the integration with next/image, which provides an optimized way to handle and optimize images in your application.
When it comes to image optimization, next/image simplifies the process by automatically optimizing and serving responsive images. It offers advanced features such as lazy loading, which ensures that images are loaded as they enter the viewport, reducing initial page load time.
The integration with next/image is straightforward. To get started, you need to import the Image component from next/image and set the src attribute to the image URL:
import Image from 'next/image'; function MyComponent() { return ( <> <Image src="/path/to/image.jpg" alt="Image description" /> </> ) }
Next.js uses its built-in optimization techniques to deliver the most optimal image size, format, and quality based on the device's screen size and resolution. This ensures that your application loads quickly while maintaining visual fidelity.
Next.js also provides a variety of configuration options to further enhance your image handling. You can specify custom sizes, pass additional attributes, and even handle fallbacks if an image fails to load.
<Image src="/path/to/image.jpg" alt="Image description" width={500} height={300} className="custom-image" onError={(e) => console.log('Image failed to load', e)} />
It's worth noting that next/image works seamlessly with popular Next.js features like static site generation (SSG) and server-side rendering (SSR). This enables you to build dynamic applications while still benefiting from the performance and optimization provided by next/image.
In conclusion, next/image simplifies image handling in Next.js applications by automating image optimization and offering advanced features like lazy loading. Its integration with Next.js is straightforward, and with a few lines of code, you can enhance your application's performance and user experience. The flexibility and configurability of next/image make it a powerful tool for handling images in your Next.js projects.
Introducing next/image
next/image is an optimized image component provided by Next.js, a popular React framework. Built with performance and SEO in mind, it offers a seamless way to handle responsive and optimized images, ensuring faster load times and a better user experience.
With next/image, you can easily handle different image formats, such as JPG, PNG, and SVG, without worrying about manually optimizing them for the web. The component automatically compresses and generates responsive versions of your images, adapting them to various screen sizes and resolutions.
One of the key advantages of next/image is its ability to lazy load images. By default, the component only loads images when they enter the viewport, minimizing the initial page load and saving bandwidth. This feature greatly enhances page performance, especially for sites with multiple images.
next/image also provides advanced accessibility features. It automatically adds the appropriate alt
text to each image based on the file name, making your site more accessible to visually impaired users and improving its SEO ranking. Additionally, it supports the inclusion of tooltips and captions to enhance the user experience further.
To use next/image, simply import it into your Next.js project and update your image tags. The component supports different image sources, including local files, remote URLs, and external providers like WordPress and Contentful. You can also specify image dimensions and easily apply custom styling using CSS-in-JS libraries like Tailwind CSS or Styled Components.
In conclusion, next/image is a powerful tool for optimizing and handling images in your Next.js applications. By incorporating it into your project, you can effortlessly enhance page loading speed, improve SEO, and provide a better user experience. Its ease of use and built-in features make it a must-have component for any modern web developer.
Benefits of next/image in Web Design
When it comes to building a visually appealing and high-performance website, the choice of image optimization and loading techniques plays a crucial role. This is where next/image comes into the picture, providing a range of benefits for web designers and developers.
One of the key advantages of using next/image is its automatic image optimization. By leveraging advanced algorithms, this tool automatically resizes and optimizes images for optimal performance, without compromising on quality. This ensures that websites load quickly, reducing bounce rates and improving user experience.
next/image also enables responsive design by providing a simple and intuitive way to handle different screen sizes and resolutions. With the built-in support for automatic image resizing, developers can easily generate and serve optimized images based on the user's viewport, resulting in a seamless experience across various devices.
In addition, next/image has an intelligent priority system for loading images. This means that critical content, such as text or important visuals, will be loaded first, while less crucial images are deferred. This prioritization not only enhances user experience but also has a positive impact on search engine optimization (SEO) by improving page load speed and perceived performance.
Another benefit of using next/image is its seamless integration with third-party image hosting services. By leveraging popular providers such as Vercel's built-in Image Optimization or Cloudinary, developers can effortlessly manage their images, including aspects like caching, scaling, and transformations.
Lastly, next/image encourages best practices in accessibility. With its support for alternative text and automatic generation of accessible image elements, it ensures that visually impaired users can access the content effectively and contributes to a more inclusive web experience.
In conclusion, next/image offers a host of benefits for web designers and developers. From automatic image optimization and responsive design to intelligent loading and accessibility support, it simplifies the process of incorporating high-quality images into websites, ultimately enhancing user experience and improving search engine rankings.
Best Practices for Implementing next/image
When it comes to optimizing images in Next.js applications, the next/image
package is a powerful solution. However, incorporating it effectively requires following some best practices. These guidelines will help you make the most out of next/image
while ensuring optimal performance and SEO.
-
Choose the Right Image Format: Selecting the appropriate image format is crucial. JPEG is ideal for photographs or complex images, while PNG is better suited for simpler graphics or images with transparency. For vector-based graphics, consider using SVG.
-
Specify Dimensions: Always define width and height attributes for
next/image
. This allows Next.js to optimize the layout and improves the loading performance. Additionally, it helps prevent layout shifting when images load. -
Leverage the
layout
Property: Use thelayout
property to control hownext/image
behaves. The most common options areresponsive
,fixed
, orintrinsic
. Applying the right layout setting ensures your images adapt seamlessly to different screen sizes and resolutions. -
Implement Responsive Images: Take advantage of
next/image
's support for different device sizes by using thesrcSet
andsizes
attributes. By specifying multiple image sources and their respective sizes, Next.js can dynamically deliver the most suitable image for each device. -
Lazily Load Images: To enhance the initial load time, enable lazy loading for your images. Use the
loading
attribute with a value oflazy
to defer loading until the image enters the viewport, improving the perceived performance. -
Consider Image Optimization: Optimize your images before uploading them. Reduce file sizes using compression tools like TinyPNG or ImageOptim. This allows for faster image loading, enhancing user experience and SEO.
-
Implement an
alt
Tag: Always include a descriptive and relevantalt
tag for each image. This improves accessibility and allows search engines to understand the image content, enhancing your SEO efforts.
By adhering to these best practices, you can ensure smooth implementation of next/image
in your Next.js projects. Remember that optimizing image loading improves overall website performance, enhances user experience, and positively impacts your SEO ranking.
Conclusion
In conclusion, 'next/image' is a powerful tool for optimizing image loading in web applications. By utilizing features like automatic image optimization, lazy loading, and responsive image delivery, developers can significantly enhance the user experience and improve overall performance.
One of the key advantages of 'next/image' is its effortless integration with Next.js, making it a seamless choice for developers working with this popular framework. The ability to specify layout optimizations and easily handle different image formats is a game-changer, allowing websites to load faster and consume fewer network resources.
Additionally, the built-in support for responsive images ensures that images adapt to various screen sizes, ensuring a consistent and visually appealing experience across devices. With 'next/image', developers can deliver the optimal version of an image tailored to each viewer's device, without compromising on quality or loading times.
Furthermore, the easy-to-use API and declarative syntax make it straightforward to incorporate 'next/image' into existing projects or start new ones. The comprehensive documentation and community support provide a strong foundation for developers to leverage the full potential of this powerful image optimization tool.
In conclusion, if you're looking to enhance the performance and loading times of your web application while delivering optimal visual experiences, 'next/image' is the solution you need. Make the most of its features, optimize your images, and give your users a delightful browsing experience.
Don't wait any longer, start using 'next/image' today and unleash the power of optimized images in your web projects!