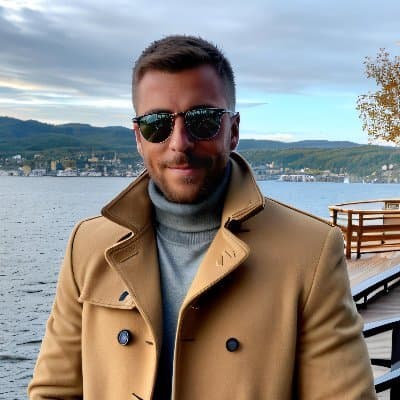
- Sep 4, 2023
- 2 min read
Unlocking the Full Potential of Web Design & Development with API Routes
Photo by Edho Pratama on Unsplash
Introduction: Understanding API Routes
API routes serve as a fundamental component in building robust and scalable applications, enabling seamless communication between different software systems. An Application Programming Interface (API) is essentially a collection of defined endpoints that allow developers to interact with an application or service, retrieving and manipulating data.
With the rising popularity of web applications and microservices architecture, mastering API routes has become crucial for modern developers. These routes are the pathways through which requests are made and responses are received, forming a bridge between client and server.
Through API routes, developers can facilitate data transmission, authentication, and various other operations. These routes provide a standardized interface for programmers to interact with, creating a layer of abstraction to simplify complex functionalities.
In this article, we will explore the concept of API routes in depth, discussing their significance and implementation strategies. We will delve into various aspects such as route handling, data serialization, and authentication methods. By the end, you will have a solid understanding of API routes and be equipped to leverage them effectively in your projects.
So, let's begin our journey into the world of API routes, empowering you to build robust and efficient software solutions.
Understanding API Routes
API routes play a crucial role in modern web development, allowing applications to communicate and exchange data. Asynchronous and versatile, API routes enable developers to create powerful, interactive web applications. In this article, we will delve into the depths of API routes, exploring their purpose, functionality, and best practices.
API routes serve as endpoints that handle incoming HTTP requests. These routes are essential for handling data retrieval, storage, and manipulation. By defining API routes, developers can create a well-structured and organized codebase, enhancing the scalability and maintainability of their applications.
One key advantage of API routes is their ability to handle both server-side and client-side logic. With API routes, developers can implement complex server-side computations, such as data validation, authentication, and database interactions. Additionally, API routes facilitate seamless integration with external APIs, enabling applications to fetch or update data from external sources.
When designing API routes, it is essential to follow best practices to ensure optimal performance and security. One such practice is to employ proper authentication and authorization mechanisms. Implementing token-based authentication or OAuth standards helps protect sensitive data and restrict access to authorized users only.
Another important aspect to consider is input validation. Validating the incoming data ensures that any operations performed on it are safe and do not compromise the application’s integrity. Failure to validate user input may lead to security vulnerabilities, such as SQL injection or cross-site scripting attacks.
Furthermore, developers should prioritize efficiency. By properly structuring API routes and minimizing unnecessary computations or database queries, applications can deliver responses rapidly, enhancing the user experience. Caching mechanisms, such as Redis, can also be utilized to store frequently accessed data and reduce response times.
In conclusion, API routes are a fundamental component of web development, facilitating seamless communication and data exchange between applications. With well-designed and optimized API routes, developers can create robust, scalable, and secure web applications. By following best practices and considering performance optimization techniques, developers can ensure their API routes provide efficient and reliable functionality, delivering a superior user experience.
Next.js Integration with API Routes
Next.js provides a seamless integration with API routes, allowing developers to build powerful server-side functionalities without the need for additional server setup. With API routes, you can easily create and handle API endpoints directly within your Next.js application.
To create an API route in Next.js, simply create a file inside the pages/api
directory. Any file in this directory will be treated as an API endpoint and mapped to a specific URL. For example, if you create a file named users.js
inside pages/api
, it will be accessible through the URL /api/users
.
API routes in Next.js are server-side rendered by default, which means that they execute on the server-side and return the result to the client. This allows you to fetch data, perform server-side computations, or interact with databases and third-party APIs securely.
Next.js API routes are powered by a built-in middleware system. This means you can handle different HTTP methods such as GET, POST, PUT, PATCH, and DELETE by exporting functions with the corresponding method names. For example, to handle a GET request, export a get
function from your API route file.
To demonstrate how API routes work, let's consider an example where we need to fetch data from an external API in our Next.js application. We can create a new API route called news.js
inside pages/api
and use the fetch
function to make an HTTP request to the external API.
export default async function handler(req, res) { const response = await fetch('https://api.example.com/news'); const news = await response.json(); res.status(200).json(news); }
In the example above, we use the fetch
function to retrieve news data from the https://api.example.com/news
endpoint. We then parse the response as JSON and send it back to the client using res.status(200).json(news)
.
It is worth mentioning that API routes support middleware functions. These functions can be used to handle authentication, authorization, or any other custom logic before executing the main API logic.
API routes in Next.js offer a powerful way to create server-side functionality within your application. With seamless integration and the ability to handle different HTTP methods, they enable developers to build robust APIs without setting up an extra server.
Benefits of API Routes in Web Design & Development
API routes offer significant advantages for web design and development projects. These routes allow developers to create custom endpoints that enable efficient data fetching, processing, and manipulation. Leveraging API routes can greatly enhance the functionality and performance of web applications. Here are some key benefits of using API routes:
1. Simplified Data Interaction
API routes simplify data interaction by providing a structured approach to retrieve and manipulate data. Developers can define custom endpoints that fetch data from various sources, such as databases or external APIs. This flexibility enables efficient and seamless integration of different data sources, reducing complexity and improving overall development time.
2. Improved Performance
By implementing API routes, web applications can optimize performance. With traditional routing, complete page reloads are often required, leading to slower loading times. On the other hand, API routes enable partial page updates through background requests, resulting in faster response times and smoother user experiences.
3. Enhanced Security
API routes provide an extra layer of security for web applications. By centralizing data handling through custom endpoints, developers can implement authentication and authorization mechanisms to validate user access. This helps safeguard sensitive information and prevent unauthorized access to data and functionalities.
4. Scalability and Agility
When designing web applications, scalability and agility are crucial factors to consider. API routes allow for modular development, enabling developers to create independent endpoints for specific functionalities. This modular approach makes it easier to add new features or modify existing ones without disrupting the overall application structure. Additionally, API routes facilitate the reuse of code, enabling developers to build upon existing functionality and improve development efficiency.
5. Better User Experience
With API routes, web applications can offer a more fluid and interactive user experience. Instead of waiting for entire page reloads, API routes enable dynamic updates of specific components, delivering real-time data changes. This can lead to faster navigation, instant data updates, and a smoother overall user experience, resulting in higher user satisfaction and engagement.
In conclusion, API routes bring numerous benefits to web design and development, including simplified data interaction, improved performance, enhanced security, scalability, agility, and better user experiences. By leveraging these advantages, developers can create robust and user-friendly web applications that meet the demands of today's increasingly dynamic digital landscape.
Implementing API Routes in Your Projects
API routes are an essential aspect of building modern web applications. They serve as the communication bridge between the frontend and the backend, allowing data retrieval and manipulation. In this section, we will explore the key steps to implement API routes in your projects.
-
Setting up a Server: First, create a server using a framework like Express.js. This will handle HTTP requests and responses, enabling the creation of API endpoints. Install the required dependencies and create a server file.
-
Defining API Routes: Design your API routes based on the functionality you want to expose. Each route corresponds to a specific HTTP method (GET, POST, PUT, DELETE) and URL path. Use concise and descriptive endpoints, adhering to RESTful principles.
-
Handling Requests: Within each route, implement the necessary logic to handle incoming requests. This involves extracting parameters from request bodies or query strings, validating input, and interacting with a database or other external services.
-
Handling Responses: After processing requests, prepare the response to send back to the client. Include meaningful status codes, such as 200 for successful requests or 404 for not found. Format the response body in JSON for easy consumption by frontend applications.
-
Middleware: Middleware functions add additional functionality to your API routes. You can authenticate requests, handle error responses, or perform request validation using middleware. Utilize existing middleware libraries or create custom ones to fit your project's requirements.
-
Testing: Thoroughly test each API route, covering various scenarios, to ensure they function correctly. Employ tools such as Postman or automated testing frameworks like Jest to verify the expected behavior of your endpoints.
-
Documentation: Document your API routes, including endpoint details, request/response examples, and any specific requirements. This documentation helps team members, external developers, and potential consumers understand how to interact with your API.
-
Security: Implement security measures to protect your API routes from unauthorized access or malicious attacks. Utilize authentication mechanisms like API keys, JSON Web Tokens, or OAuth2. Additionally, validate user input to prevent common vulnerabilities like SQL injection or cross-site scripting attacks.
By following these steps, you can effectively implement API routes in your projects. They serve as the backbone for your application's data flow, enabling seamless communication between the frontend and backend.
Conclusion
In conclusion, API routes are an essential component of modern web development. They provide a structured and efficient way to handle requests and responses between servers and clients. By defining routes and endpoints, developers can easily organize and manage their APIs, enabling seamless communication between different systems and applications.
Throughout this article, we discussed the importance of API routes and how they contribute to the overall functionality and performance of web applications. We explored how they allow developers to implement RESTful principles, enabling resource-based interactions and clear separation of concerns.
API routes offer a flexible and scalable approach to building APIs, making it easier to handle different types of requests such as GET, POST, PUT, and DELETE. Moreover, they provide a secure way to authenticate and authorize access, ensuring that sensitive information remains protected.
To effectively utilize API routes, it is crucial to follow best practices and adhere to standard conventions. By properly documenting endpoints, leveraging versioning, and using appropriate response codes, developers can enhance the usability and maintainability of their APIs.
In conclusion, API routes empower developers to create robust and versatile web applications. They enable seamless communication between clients and servers, offering efficient data retrieval and manipulation. By implementing well-designed API routes, developers can streamline development processes, improve performance, and enhance user experiences.
Are you ready to harness the power of API routes in your own projects? Start exploring and experimenting with different frameworks and libraries that support API routing, and unleash the full potential of your web applications. Happy coding!