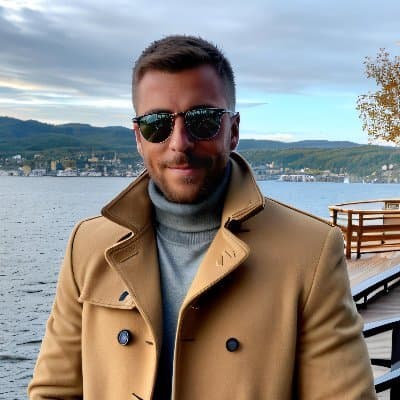
- Sep 5, 2023
- 2 min read
Maximize Your Web Speed: Dynamic Imports in Web Design & Development
Photo by Krisztian Tabori on Unsplash
Introduction to Dynamic Imports
Dynamic imports represent a JavaScript module loading feature that developers leverage to import functions, objects, or values into their programs directly. They conserve resources and enhance application performance by enabling code-splitting—an approach where code is broken into chunks that can be loaded on demand. This dynamic code loading feature significantly speeds up the initial load time of your web applications.
Traditionally, static import
statements orchestrate a top-of-file operation, loading all objects at the start of the program. However, dynamic imports introduce a game-changing paradigm. They let you import modules dynamically as functions within your code, allowing asynchronous loading and an overall streamlined application performance.
But what does it mean to import modules dynamically? What benefits does this technology offer, and how does it impact the way developers code? This article will dive into these questions, providing an in-depth examination of dynamic imports, their usage, benefits, and role in modern web development. Whether you're a seasoned developer or someone just stepping into the world of JavaScript, this exploration of dynamic imports is sure to provide valuable insights.
Experience the evolution in JavaScript module loading with dynamic imports, a significant step towards efficient coding and optimized web application.
The Importance of Fast Site Performance
Fast site performance is a crucial goal for every web developer. In an age where users expect instantaneous results, a delay of even few seconds can lead to customer dissatisfaction and potential business loss. Optimization of your site's performance is essential for ensuring user engagement, satisfaction, and ultimately, conversion.
One of the key ways to accelerate site performance is through dynamic imports. Dynamic imports allow code splitting, a feature made possible by modern bundlers like Webpack and Parcel. This technique essentially allows you to split your JavaScript code into separate chunks, which can be loaded on demand. In this way, a user only loads the code they need at any given moment, rather than loading all code at once. This ensures faster initial load times and an overall superior user experience.
As search engines like Google continue to emphasize site speed in their ranking algorithms, the importance of fast site performance has never been greater. A high-performance site not only delights users but also contributes towards better search engine rankings, resulting in increased organic traffic.
One major advantage of dynamic imports is that they allow for optimization at a granular level. For instance, you can selectively load heavyweight libraries only on pages where they are needed, thereby minimizing unnecessary load on your servers and on the user's device.
The asynchronous nature of dynamic imports has one further significant benefit: it promotes more effective utilization of browser's resources. By parallelizing tasks that can be performed independently, dynamic imports facilitate a smoother and more responsive user experience, especially on single-page applications (SPAs).
In sum, fast site performance plays a strategic role in maintaining user satisfaction and engagement, improving SEO rankings, and optimizing resource usage. Implementing techniques like dynamic imports is an effective way to cater to these needs.
Dynamic Imports: The Role in Code Splitting
Dynamic Imports play a crucial role in the practice of code splitting, which can significantly optimize your app's performance. Employing dynamic imports allows for modules to be loaded on-demand. The process enhances user experience by loading only the necessary chunks of code when a specific function is initiated.
Code splitting involves dividing a significant JavaScript bundle into smaller chunks that can be lazily loaded on demand. The process is done instead of loading the entire JavaScript file in one go. Dynamic imports stand out as an essential tool in making code splitting work.
Using dynamic imports in JavaScript, modules are imported as functions instead of statements. Furthermore, these import() calls return promises, ensuring reliable asynchronous behavior. Instead of loading all modules at the parse time, import() syntax loads them at runtime. This approach significantly reduces the amount of JavaScript that needs to be transferred, parsed, and evaluated by the browser.
Consider a scenario where a significant part of your Javascript functionality is only required under specific conditions, say, user interaction. Dynamic imports will essentially "lazy-load" these parts, which are not necessary at the initial page load. Therefore, the smallest amount of code gets shipped to the user initially, thereby significantly improving your application's loading performance.
However, remember that code splitting is not a silver bullet. While it can help to reduce the amount of JavaScript loaded up front, it can sometimes lead to more network requests. Therefore, thoughtful considerations should be made when deciding what to split and when to split.
Dynamic Imports not only brings code splitting to the next level but also offers developers a more granular control of module loading, which can, ultimately, make applications run more efficiently.
Implementing Dynamic Imports in Web Development
Dynamic imports, an integral component of modern Web Development, introduce the flexibility of loading modules on demand. In comparison to static imports, dynamic imports offer a more efficient approach by reducing initial load time and potentially improving overall application performance.
In ES6, you encounter the import()
syntax, which is a function-like method that returns a Promise. This Promise, when resolved, returns a module object.
import('./module.js') .then((module) => { // Use module's default export console.log(module.default); }) .catch((err) => { // Handle error console.log(err); });
In the above example, module.js
is dynamically imported. The module’s default export could then be used within the then()
block once the Promise has resolved.
Webpack, a popular module bundler, natively supports dynamic imports. Its code-splitting feature coupled with import()
can partition applications into smaller chunks that load on demand.
React, a widely used JavaScript library, also takes advantage of dynamic imports, especially for component lazy loading. React's built-in React.lazy()
function supplements the use of import()
, allowing components to be loaded dynamically.
const DynamicComponent = React.lazy(() => import('./DynamicComponent')); // DynamicComponent can be used like regular components within <Suspense> fence
While dynamic imports may not be suited to every application's needs, they offer immense benefits in the correct context. Precise implementation aids application optimization along with creating a fluid user interface, thereby improving the user experience.
Strategies for Dynamic Imports and Faster Site Performance
The concept of dynamic imports allows for efficient management of web module loading. Below are several strategies to increase site performance through dynamic imports:
Prefetching and Preloading
Prefetching and preloading are great tools for faster site performance. Prefetching quietly loads resources when the site is idle, ready for future navigation. It predicts what resources might be needed and loads them in advance. Preloading, on the other hand, is directive to the browser about resources required for the current page.
Code Splitting
Code splitting is another effective strategy. It breaks up your code into smaller, manageable chunks. These chunks are then loaded on demand, reducing initial load and making site interaction faster. The dynamic import()
function makes this possible and easy to manage.
Tree Shaking
Tree shaking, this concept allows for the removal of unused code from the final bundle. By keeping the bundle size small, loading times are significantly reduced. Proper configuration should be done for optimum tree shaking outcomes.
Lazy Loading
Lazy loading enhances site performance by loading content only when it's needed. The user's scrolling behavior triggers this loading. It can be applied to images, scripts, or any content that weighs down site speed.
Remember, faster sites not only provide a better user experience but also perform better in terms of SEO. As we leverage the power of dynamic imports in improving site performance, we optimize for search engine visibility.
To conclude, these four strategies – when employed correctly – in conjunction with dynamic imports deliver efficient and speedy site loading, enhancing both user satisfaction and search engine rankings.
Remember, dynamic imports not only facilitate a clean codebase, but also gear your site up for optimum performance.
You might also want to consider using HTTP/2 for further speed improvements. It allows for parallel loading of modules, reducing the number of server requests and leading to faster page loads.
Remember, each site is different. Utilize these strategies thoughtfully, test regularly, and watch the site performance soar.
Real-World Examples of Dynamic Imports
Understanding the application of dynamic imports in real-world scenarios proves immensely helpful. Dynamic imports come in handy when working with modules and components that don't always have to be loaded. They allow users to defer loading certain parts of JavaScript until they're needed, conserving resources and enhancing performance.
Example 1: E-commerce website
An e-commerce website may have hundreds of product descriptions, images, reviews, ratings, etc. Dynamic imports can be used to load specific product details only when a user clicks on a product.
button.addEventListener("click", event => { import("./product-details.js") .then(module => { const productDetails = module.default; productDetails.render(); }) .catch(error => console.log(error)); });
Example 2: Translating UI Text
Say you have an application with text translated into multiple languages. Loading every language version upfront would significantly slow the app down. By utilizing dynamic imports, you only load the language pack specific to the user’s location.
let userLang = navigator.language || 'en'; import(`./texts-${userLang}.js`) .then(texts => { document.getElementById('welcome').textContent = texts.welcome; }) .catch(error => console.log(error));
Example 3: Lazy Loading of Features
Features not required immediately, such as chatbots, can be lazy-loaded to not burden the initial JavaScript payload. With dynamic imports, these features are loaded only when needed, improving end-user experience.
let chatIcon = document.getElementById("chat-icon"); chatIcon.addEventListener("click", event => { import("./chatbot.js") .then(chatbot => { chatbot.init(); }) .catch(error => console.log(error)); });
These examples illustrate how dynamic imports offer more control and flexibility, enhancing overall application efficiency and user experience.
Conclusion
Throughout this article, we've discussed the concept of dynamic imports in terms of their power, flexibility, and the possibilities they offer. Dynamic imports, an integral part of modern JavaScript, have revolutionized the development process, making it efficient and seamless. We've studied how dynamic imports help load modules dynamically based on certain conditions, improving load times and app performance.
Scenarios requiring code on-demand like loading widgets, Graphs, Maps, etc., or loading additional scripts based on user actions have immensely benefitted by utilizing dynamic imports. Investing in understanding and implementing this feature can drastically improve user experience by reducing initial load time and making your applications faster and more responsive.
Furthermore, the utilization of dynamic imports gives developers more control to fine-tune their apps, deciding when and what parts of their code should be loaded. This ensures that users are not made to load unnecessary scripts, optimizing the overall interaction process.
Javascript's provision for Promise interface with dynamic imports adds to the flexibility, letting developers code asynchronously, ensuring non-blocking behavior of the application, and making way for efficient error handling.
To conclude, the use of dynamic imports is an essential skill for any modern-day JavaScript developer. The capabilities it provides are invaluable for developing fast, efficient, and user-friendly applications. Now that you have a good understanding of dynamic imports, it's time to put this knowledge to work in your projects. Start optimizing your code and create applications that stand out, all the while providing the best user experience. Every bit of effort towards mastering dynamic imports will be a step towards becoming a better, more professional developer. So, go ahead and harness the power of dynamic imports!