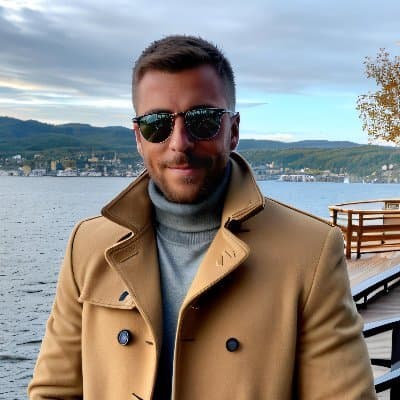
- Sep 4, 2023
- 2 min read
The Ultimate Guide to Vue Router: Seamless Web Design & Development Integration
Photo by Edho Pratama on Unsplash
Introduction
Vue Router is a powerful routing library for Vue.js, the progressive JavaScript framework. With Vue Router, you can easily handle navigation in your single-page applications, allowing users to move between different views and components seamlessly.
Routing is an essential aspect of modern web development, as it enables the creation of dynamic, interactive user experiences. Vue Router empowers developers to build robust, scalable applications by providing a clean and efficient way to manage the application's state and handle client-side navigation.
This article explores the various features and capabilities of Vue Router, highlighting its importance in the Vue.js ecosystem. We will delve into key concepts such as route configuration, dynamic routing, nested routes, and navigation guards. By understanding these concepts, you will be able to leverage Vue Router to create smooth and responsive web applications.
By the end of this article, you will have gained a comprehensive understanding of Vue Router, enabling you to harness its full potential in your Vue.js projects. So, let's dive in and explore the powerful capabilities of Vue Router, enhancing user experience and taking your Vue applications to new heights.
Understanding Vue Router and its role in Web Design & Development
Vue Router is a crucial component of Vue.js, a progressive JavaScript framework used for building user interfaces. It provides a powerful routing system that enables developers to create single-page applications with ease. So, what exactly is Vue Router and what role does it play in web design and development?
At its core, Vue Router allows for the creation of multiple pages within a single web application. This is accomplished by mapping each page to a specific URL, resulting in a smooth and seamless user experience. By leveraging Vue Router, developers can build dynamic, responsive websites that offer enhanced navigation and intuitive user interactions.
One of the key advantages of using Vue Router is its ability to handle client-side routing. Instead of relying on server requests for each page change, Vue Router dynamically updates the content on the client side. This feature significantly improves performance and reduces the load on the server, resulting in faster page loads and a more responsive user interface.
Furthermore, Vue Router offers powerful features such as nested routes, route parameters, and route meta fields. Nested routes allow for the creation of complex page structures, where components can be nested within one another. Route parameters enable the passing of dynamic data through URLs, allowing for more personalized and customized pages. Additionally, route meta fields allow for the definition of additional information associated with each route, such as authentication requirements or page titles.
From a web design and development perspective, Vue Router simplifies the process of creating and managing routes within a Vue.js application. Its declarative syntax, which closely resembles HTML, makes it intuitive to work with and allows for easy navigation between pages. Additionally, Vue Router integrates seamlessly with other Vue.js components, enabling efficient development workflows and fostering code reusability.
In conclusion, Vue Router plays a vital role in web design and development by providing a robust routing system for Vue.js applications. Its ability to handle client-side routing, coupled with its powerful features, makes it a valuable tool for creating dynamic and responsive websites. By utilizing Vue Router, developers can deliver seamless navigation experiences to users while optimizing performance and enhancing the overall quality of their web applications.
Setting up Vue.js Integration with Vue Router
Vue Router is a powerful routing library for Vue.js applications. It allows us to build single-page applications with multiple views and navigation capabilities. To integrate Vue Router into a Vue.js project, follow these steps:
-
Install Vue Router: Use npm or yarn to install Vue Router package by running the following command:
npm install vue-router
oryarn add vue-router
. -
Create a Router File: In your project's directory, create a new file called
router.js
orindex.js
. Import Vue and Vue Router, then create a new Router instance usingVueRouter()
. -
Declare Routes: Inside your Router file, declare the routes for your application. Each route should have a corresponding component. Use the
path
property to define the URL path and thecomponent
property to map the route to a Vue component. -
Import Router into Main.js: In your
main.js
file, import therouter.js
file and add the router instance to the Vue instance.
import Vue from 'vue'; import App from './App.vue'; import router from './router'; new Vue({ router, render: h => h(App) }).$mount('#app');
-
Set up Router-View: In your main App component, add the
<router-view></router-view>
tag. This tag will render the appropriate component based on the current route. -
Navigation: To navigate between routes, use the
<router-link>
component. Set theto
attribute to the desired route path. Alternatively, you can use therouter.push()
method programmatically.
Vue Router offers advanced features like nested routes, route parameters, and route guards. Refer to the official documentation for more details.
Optimizing Vue Router integration with Vue.js will improve your application's performance and user experience, resulting in better search engine rankings. By following these steps, you can efficiently set up Vue Router in your Vue.js project.
Creating Routes and Navigation
Vue Router is a powerful tool for building single-page applications with Vue.js. One of its key features is the ability to create routes and handle navigation within your application.
To create routes, you first need to define them in your router configuration. This can be done using the routes
option, where each route is an object with a path
and a component
. The path
defines the URL path that should match the route, while the component
specifies the Vue component to render when the route is accessed.
Here's an example of defining routes in Vue Router:
const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, { path: '/contact', component: Contact } ]
Once you have defined your routes, you need to add the router to your Vue application by creating an instance of Vue Router and passing in the routes configuration:
const router = new VueRouter({ routes }) new Vue({ router }).$mount('#app')
With the routes set up, you can now navigate between them using the <router-link>
component. This component is used to create links to different routes in your application. It automatically updates the URL and renders the appropriate component when clicked.
<router-link to="/">Home</router-link> <router-link to="/about">About</router-link> <router-link to="/contact">Contact</router-link>
To display the current route's component, you need to add a <router-view>
component to your Vue template. This component acts as a placeholder that renders the current route's component.
<router-view></router-view>
Vue Router also provides additional features like nested routes, route parameters, and route guards. These advanced features allow you to create more complex navigation patterns and add additional functionality to your routes.
In conclusion, Vue Router offers a simple and intuitive way to create routes and handle navigation in Vue.js applications. By defining routes, using the <router-link>
component, and adding a <router-view>
component, you can easily create a seamless navigation experience for your users. Explore the documentation for more detailed information on the capabilities of Vue Router.
228 words.
Advanced Vue Router Features for Web Design & Development
Vue Router is a powerful routing library for Vue.js that allows us to build single-page applications with ease. While it offers the basics of routing, it also provides advanced features that can greatly enhance the development process. In this section, we will explore some of these advanced features and how they can benefit web designers and developers.
-
Lazy Loading: With large applications, it's crucial to optimize performance by loading components only when needed. Vue Router allows lazy loading of routes, which means that the code for a particular route is loaded on-demand, reducing initial page load time and improving the user experience.
-
Nested Routes: To create complex applications with multiple levels of nested routes, Vue Router offers the ability to define child routes. This allows for a clean and organized project structure, making it easier to manage and navigate through various pages and components.
-
Route Transitions: Adding animations and transitions between routes can greatly enhance the visual appeal of an application. Vue Router provides built-in support for route transitions, allowing developers to create smooth and engaging page transitions effortlessly.
-
Custom Routing Logic: Sometimes, we may need to implement custom routing logic based on certain conditions or user roles. Vue Router allows us to define dynamic routes with custom logic, enabling us to handle authentication, authorization, and other complex routing scenarios effectively.
-
Navigation Guards: Vue Router includes navigation guards, which are hooks that allow us to control and manage navigation behavior. These guards can be used to authenticate routes, handle redirects, and perform other actions before entering or leaving a route.
-
Meta Tags and SEO: To improve the SEO of our single-page applications, Vue Router provides support for adding meta tags to each route. This allows for better indexing by search engines, resulting in improved visibility and higher rankings in search results.
With these advanced features, Vue Router empowers web designers and developers to create robust, performant, and visually appealing single-page applications. By leveraging lazy loading, nested routes, route transitions, custom logic, navigation guards, and SEO optimization, developers can deliver exceptional user experiences while maintaining a clean and organized codebase.
Optimizing Performance with Vue Router
Vue Router is a powerful routing library for Vue.js that allows you to create single-page applications with ease. However, as your application grows, it is crucial to optimize the performance of your routing setup to ensure a smooth user experience. Here are some tips to help you optimize performance with Vue Router.
1. Lazy Loading Routes
One of the most effective ways to improve the performance of your Vue Router is by implementing lazy loading routes. This means that instead of loading all the routes and their associated components upfront, you can dynamically load them only when needed. By lazily loading routes, you can reduce the initial bundle size and improve the overall loading time.
2. Code Splitting
Along with lazy loading routes, code splitting is another technique that can significantly enhance performance. Code splitting allows you to split your application code into smaller chunks, which can be loaded separately as needed. This ensures that users only download the code required for the current route, reducing the initial load time and improving performance.
3. Route-Based Code Splitting
To take code splitting a step further, you can implement route-based code splitting. This technique enables you to split your code based on individual routes, so each route's components and dependencies are only loaded when the route is accessed. This can greatly improve performance by further reducing the initial bundle size and loading time.
4. Prefetching Data
To further optimize performance, you can prefetch data for upcoming routes. Vue Router provides a prefetch method that allows you to load data in the background while the user is interacting with the current page. By prefetching data for upcoming routes, you can ensure that the data is readily available when the user navigates to a new route, providing a seamless experience.
5. Route-Level Chunking
In addition to code splitting, you can take advantage of route-level chunking to optimize performance. Route-level chunking allows you to group related components and dependencies into separate chunks, ensuring that only the necessary code is loaded for each route. This can significantly reduce the size of the initial bundle and improve loading speed.
Conclusion
By implementing these optimization techniques, you can enhance the performance of your Vue Router application. Lazy loading routes, code splitting, route-based code splitting, prefetching data, and route-level chunking are all effective strategies that can significantly improve loading times and provide a better user experience. Consider implementing these techniques based on your application's specific needs to ensure optimal performance.
Conclusion
In conclusion, Vue Router is a powerful tool for creating single-page applications with Vue.js. Its intuitive API and seamless integration with the Vue ecosystem make it a go-to choice for developers. Through its routing capabilities, Vue Router enables the creation of complex navigation systems and enhances user experience.
One of the key advantages of Vue Router is its ability to handle dynamic route matching, allowing for dynamic content loading without refreshing the entire page. This improves performance and provides a smooth and interactive user experience. Additionally, Vue Router offers advanced features such as nested routes, named views, and route meta fields, further enhancing the flexibility and modularity of applications.
With its comprehensive documentation and active community support, Vue Router is easy to learn and implement in both small and large-scale projects. Whether you're a beginner or an experienced developer, Vue Router empowers you to create robust and scalable applications.
In conclusion, if you're looking for a flexible and efficient routing solution for your Vue.js applications, Vue Router is the way to go. Start exploring the power of Vue Router today and take your Vue.js development to the next level.